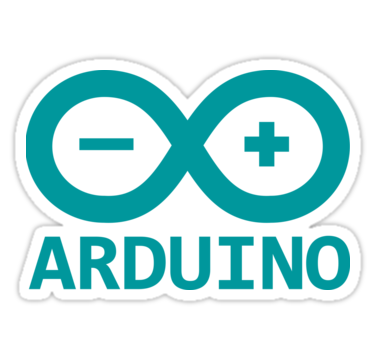
In this post I will teach you how to receive data from arduino.
You need to have Arduino IDE, if you don’t have arduino IDE you can download of here.
The firs step is write the next code, the code has comments:
//Global variable int num; void setup() { //Initialization of serial port in 9600 baud Serial.begin(9600); //Global variable num in 0 num=0; } void loop() { // num variable add 1 number in this loop num=num+1; //write the num variable Serial.println(num); //and make a second delay delay(1000); }
You should have somethin like this, and you press in arrow button to past the code to arduino card and check what is the com port, the majority times it is com3:
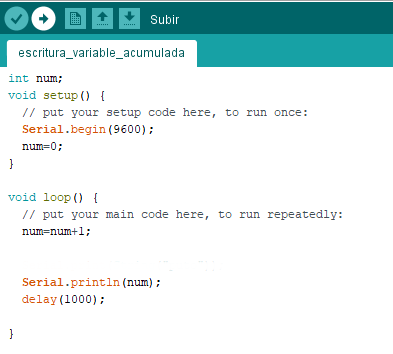
Now, you shall open visual studio and create a Windows Forms project, when the project is created you add a textbox control with the name txtSomething and you form should look like this:
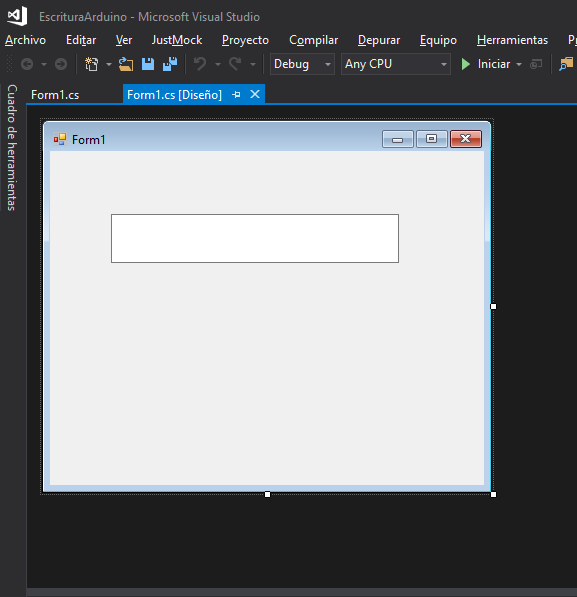
Now, you key F7 to go to view code, and write the next code, the code has comments:
public partial class Form1 : Form { //object serialport to listen usb System.IO.Ports.SerialPort Port; //variable to check if arduino is connect bool IsClosed = false; public Form1() { InitializeComponent(); //configuration of arduino, you check if com3 is the port correct, //in arduino ide you can make it Port = new System.IO.Ports.SerialPort(); Port.PortName = "COM3"; Port.BaudRate = 9600; Port.ReadTimeout = 500; try { Port.Open(); } catch { } } private void Form1_Load(object sender, EventArgs e) { //A Thread to listen forever the serial port Thread Hilo = new Thread(ListenSerial); Hilo.Start(); } private void ListenSerial() { while (!IsClosed) { try { //read to data from arduino string AString = Port.ReadLine(); //write the data in something textbox txtSomething.Invoke(new MethodInvoker( delegate { txtSomething.Text = AString; } )); } catch { } } } private void Form1_FormClosed(object sender, FormClosedEventArgs e) { //when the form will be closed this line close the serial port IsClosed = true; if (Port.IsOpen) Port.Close(); } }
Ok, with that you should receive data in your textbox.
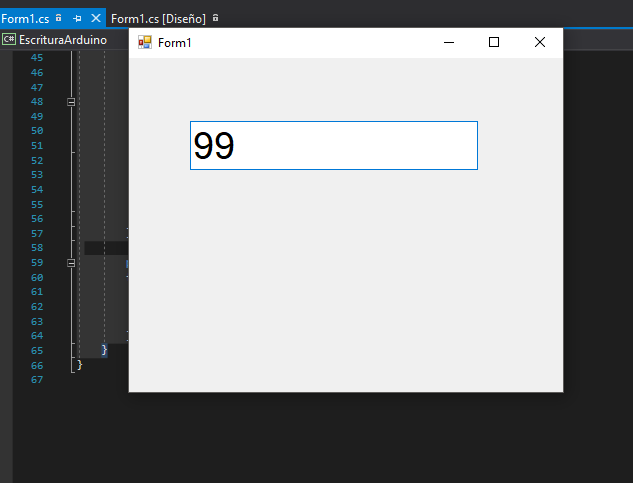
I hope you find it useful.